08. Filters & Interceptors. CDI . Conclusions
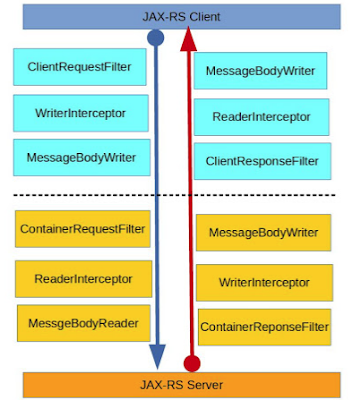
As Java Brains says we should distinguish several components that can be used both from client and server in order intercept the request or response and modify them. 1 Interceptors, Filters and MessageBody Interceptors manipulate entities ( body ) in input and output streams while filters only manipulate the request or response params like headers , URIs etc There are 2 kinds of interceptors ReaderInterceptor and WriterInterceptor . To create an interceptor, you should implement one of the 2 interfaces. One of the possible use is to GZip the body for slow communications.There are 2 kinds too of filters Container RequestFilters and ContainerResponseFilters one for the request and the other for the response. the basic usages are Logging, security.. Filters and interceptors can work both on the client and server side. On the client side, we have these filters: ClientRequestFilter and ClientResponseFilter . We also have MessageBodyReader and MessageBodyWriter for the bo